处理优化。
正在显示
预定系统/Base/DeviceA-ShareScreen.png
0 → 100644
767.3 KB
预定系统/Base/DeviceB-ShareScreen.png
0 → 100644
613.8 KB




此差异已折叠。
此差异已折叠。




此差异已折叠。



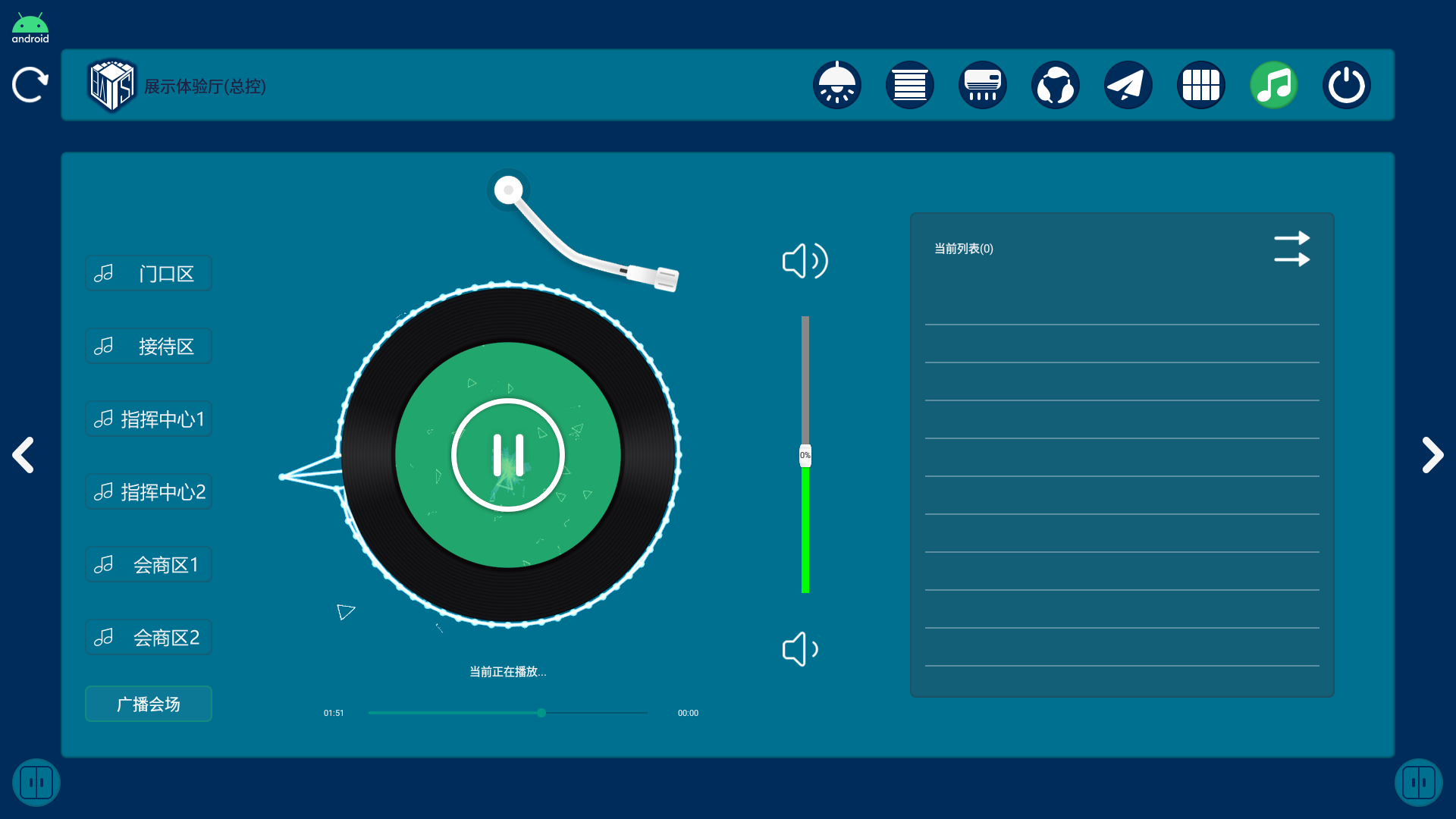






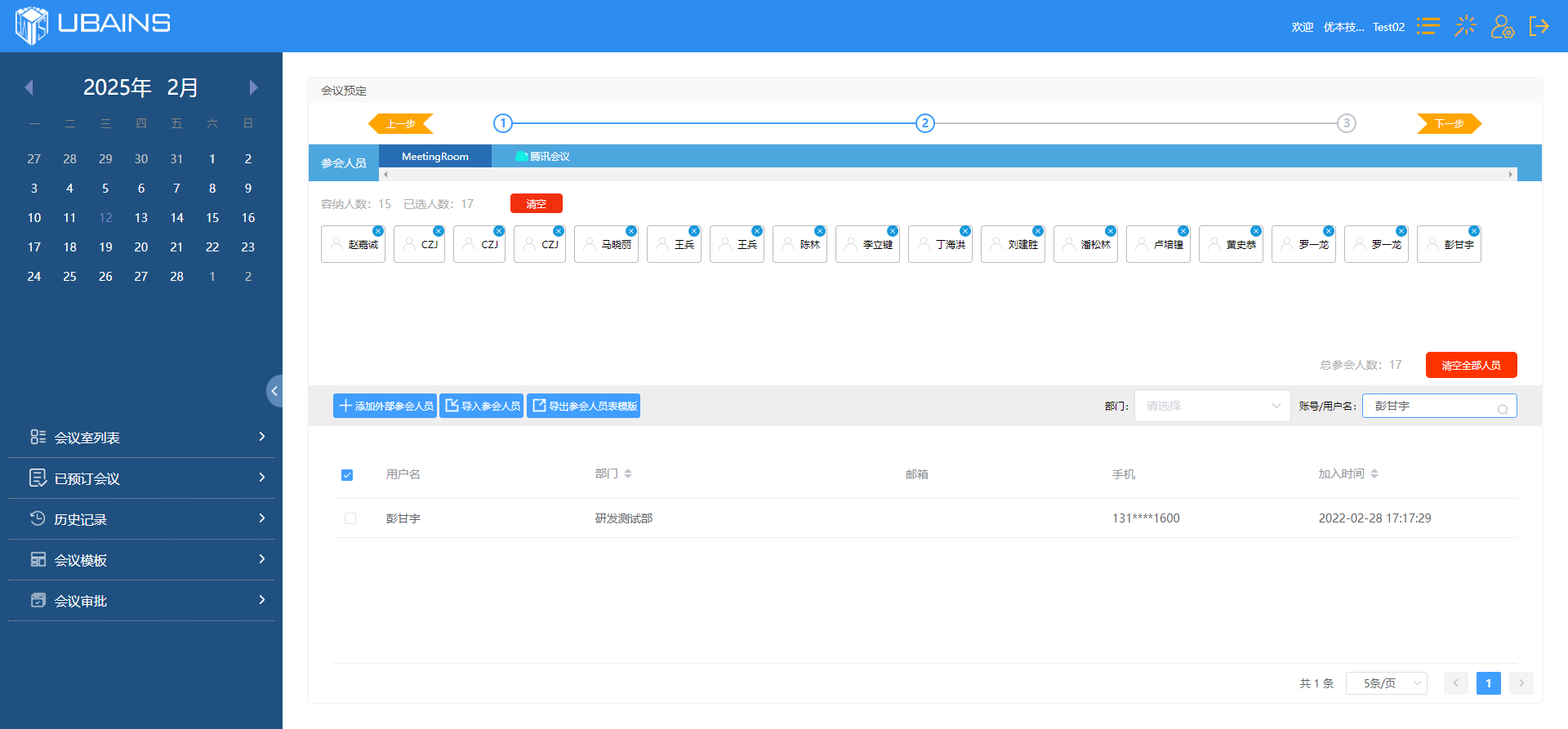


此差异已折叠。







此差异已折叠。





























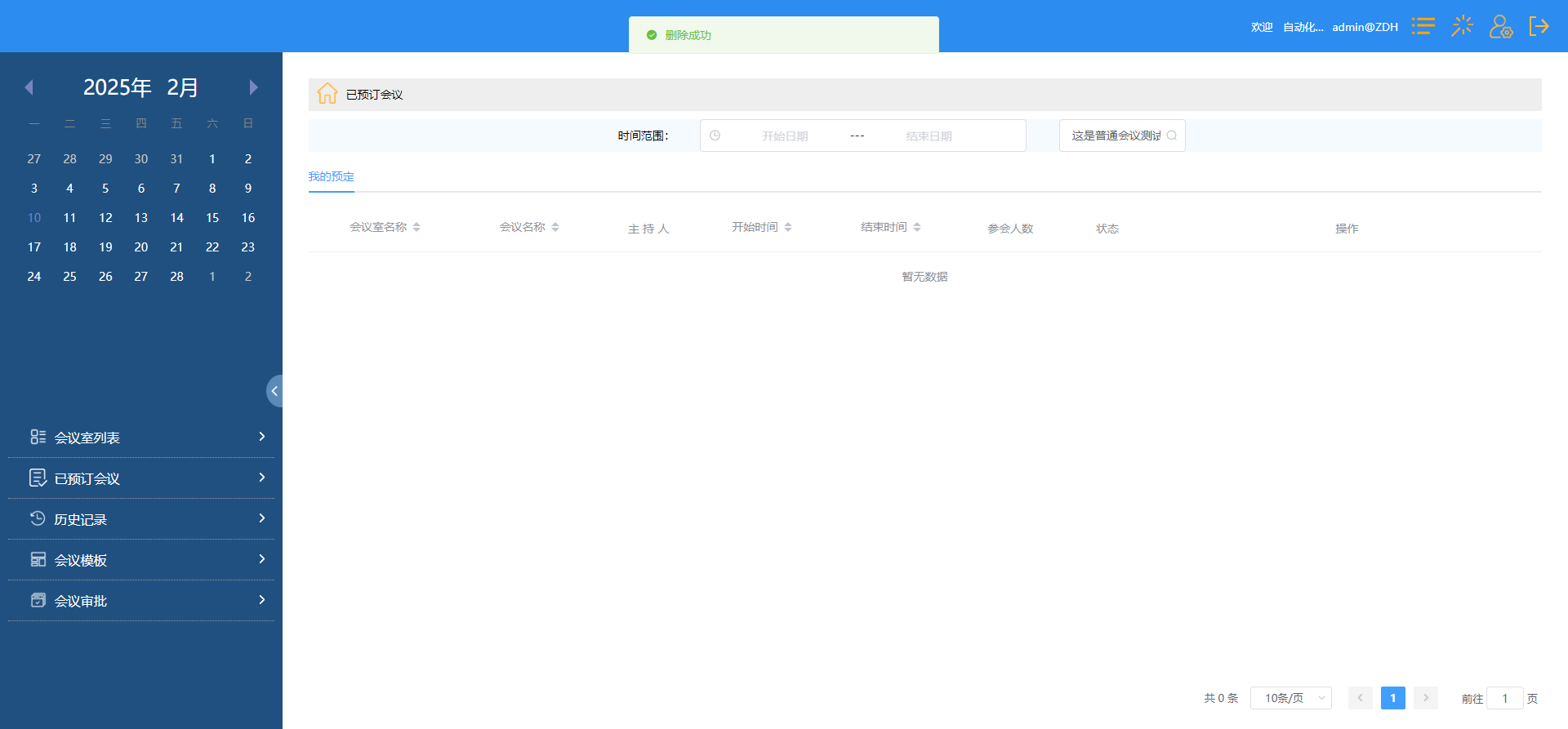




767.3 KB
613.8 KB
405.2 KB | W: | H:
99.0 KB | W: | H:
104.3 KB | W: | H:
99.0 KB | W: | H:
349.2 KB | W: | H:
349.9 KB | W: | H:
460.2 KB | W: | H:
453.4 KB | W: | H:
93.0 KB | W: | H:
93.8 KB | W: | H:
474.2 KB | W: | H:
455.9 KB | W: | H:
475.4 KB | W: | H:
455.8 KB | W: | H:
471.3 KB | W: | H:
455.2 KB | W: | H:
216.7 KB | W: | H:
222.2 KB | W: | H:
355.4 KB | W: | H:
357.0 KB | W: | H:
203.8 KB | W: | H:
203.7 KB | W: | H:
225.1 KB | W: | H:
225.2 KB | W: | H:
66.4 KB | W: | H:
66.4 KB | W: | H:
61.6 KB | W: | H:
61.6 KB | W: | H:
64.9 KB | W: | H:
64.6 KB | W: | H:
56.3 KB | W: | H:
56.3 KB | W: | H:
56.6 KB | W: | H:
56.6 KB | W: | H:
76.3 KB | W: | H:
76.3 KB | W: | H:
77.0 KB | W: | H:
77.0 KB | W: | H:
103.5 KB | W: | H:
103.7 KB | W: | H:
199.8 KB | W: | H:
199.7 KB | W: | H:
79.1 KB | W: | H:
79.3 KB | W: | H:
198.5 KB | W: | H:
198.3 KB | W: | H:
112.0 KB | W: | H:
110.5 KB | W: | H:
83.0 KB | W: | H:
83.1 KB | W: | H:
133.7 KB | W: | H:
134.0 KB | W: | H:
144.7 KB | W: | H:
144.6 KB | W: | H:
146.1 KB | W: | H:
145.8 KB | W: | H:
75.7 KB | W: | H:
75.7 KB | W: | H:
63.5 KB | W: | H:
63.5 KB | W: | H:
402.9 KB | W: | H:
101.0 KB | W: | H:
57.2 KB | W: | H:
57.4 KB | W: | H:
59.1 KB | W: | H:
59.1 KB | W: | H:
81.2 KB | W: | H:
68.5 KB | W: | H:
79.7 KB | W: | H:
68.4 KB | W: | H:
79.7 KB | W: | H:
68.4 KB | W: | H:
90.8 KB | W: | H:
90.4 KB | W: | H:
55.0 KB | W: | H:
55.2 KB | W: | H:
64.1 KB | W: | H:
64.1 KB | W: | H:
92.1 KB | W: | H:
105.9 KB | W: | H:
52.5 KB | W: | H:
52.5 KB | W: | H:
52.4 KB | W: | H:
52.4 KB | W: | H:
108.1 KB | W: | H:
107.8 KB | W: | H:
63.8 KB | W: | H:
63.7 KB | W: | H:
73.6 KB | W: | H:
79.4 KB | W: | H:
82.8 KB | W: | H:
82.4 KB | W: | H:
52.4 KB | W: | H:
52.4 KB | W: | H:
108.0 KB | W: | H:
107.6 KB | W: | H:
81.5 KB | W: | H:
81.6 KB | W: | H:
68.4 KB | W: | H:
68.4 KB | W: | H:
74.1 KB | W: | H:
74.2 KB | W: | H:
75.6 KB | W: | H:
76.1 KB | W: | H:
68.5 KB | W: | H:
68.5 KB | W: | H:
74.1 KB | W: | H:
74.3 KB | W: | H:
75.0 KB | W: | H:
75.5 KB | W: | H:
68.4 KB | W: | H:
68.4 KB | W: | H:
57.3 KB | W: | H:
57.5 KB | W: | H:
51.6 KB | W: | H:
51.6 KB | W: | H:
51.6 KB | W: | H:
51.6 KB | W: | H:
51.6 KB | W: | H:
51.6 KB | W: | H:
74.0 KB | W: | H:
74.0 KB | W: | H:
74.0 KB | W: | H:
74.0 KB | W: | H:
73.5 KB | W: | H:
74.0 KB | W: | H: